How Builders Can Make improvements to Their Debugging Capabilities By Gustavo Woltmann
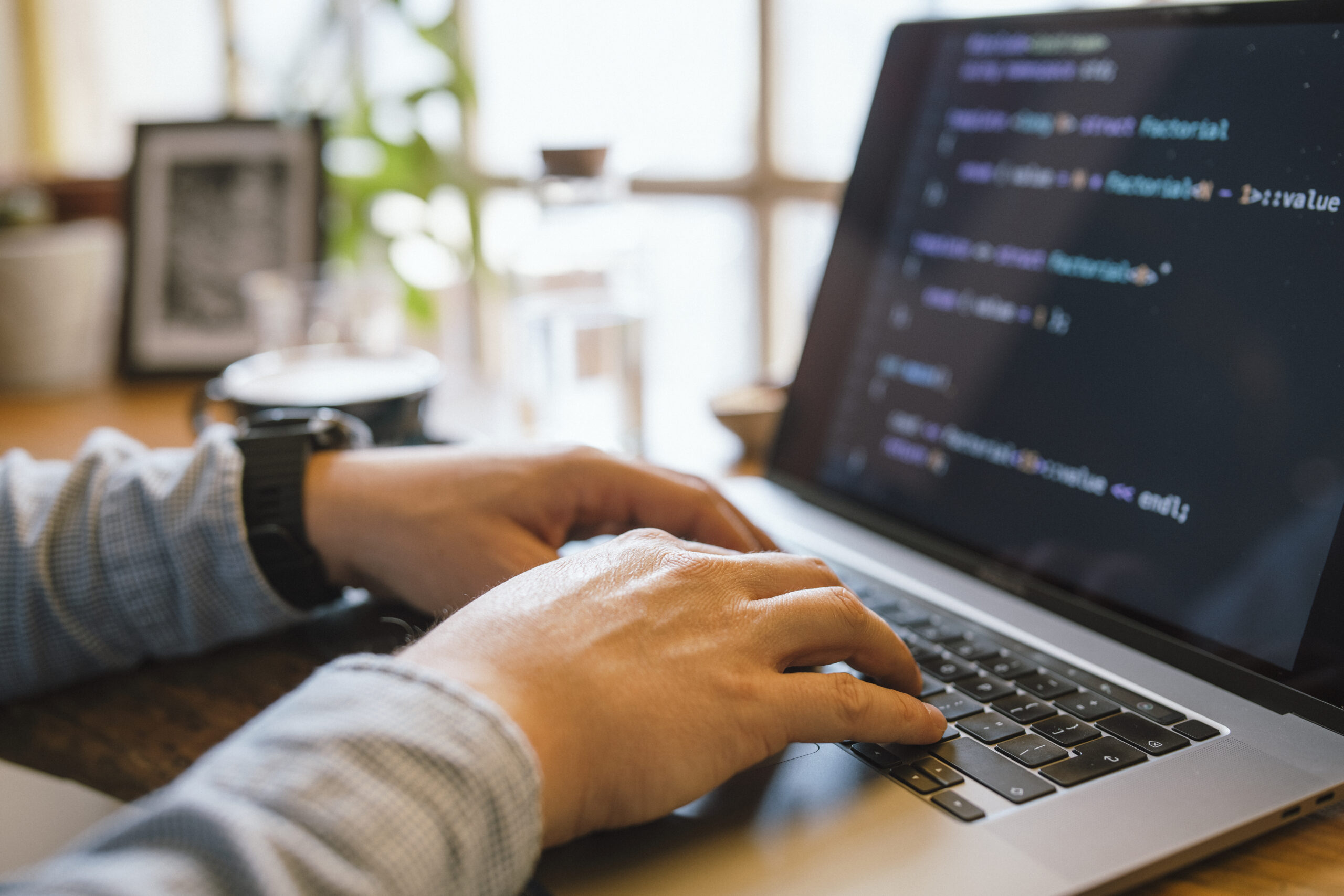
Debugging is one of the most vital — nonetheless frequently disregarded — techniques inside of a developer’s toolkit. It's not just about fixing broken code; it’s about understanding how and why items go Improper, and learning to Assume methodically to unravel issues proficiently. No matter if you are a rookie or simply a seasoned developer, sharpening your debugging skills can help you save hrs of irritation and drastically help your efficiency. Here are a number of techniques to help you developers degree up their debugging recreation by me, Gustavo Woltmann.
Grasp Your Instruments
One of several quickest techniques developers can elevate their debugging expertise is by mastering the equipment they use every single day. Even though composing code is one particular Component of growth, figuring out the way to communicate with it successfully during execution is Similarly significant. Present day growth environments arrive equipped with potent debugging capabilities — but many builders only scratch the surface area of what these resources can perform.
Acquire, by way of example, an Integrated Improvement Environment (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These applications assist you to established breakpoints, inspect the worth of variables at runtime, stage through code line by line, and even modify code to the fly. When utilised effectively, they Permit you to notice precisely how your code behaves during execution, which happens to be priceless for tracking down elusive bugs.
Browser developer instruments, for example Chrome DevTools, are indispensable for front-conclude developers. They help you inspect the DOM, keep an eye on network requests, perspective true-time overall performance metrics, and debug JavaScript from the browser. Mastering the console, sources, and network tabs can turn annoying UI issues into manageable responsibilities.
For backend or system-degree builders, resources like GDB (GNU Debugger), Valgrind, or LLDB present deep control in excess of operating procedures and memory administration. Understanding these applications might have a steeper Mastering curve but pays off when debugging performance troubles, memory leaks, or segmentation faults.
Further than your IDE or debugger, come to be comfy with Model control techniques like Git to be aware of code record, discover the exact second bugs ended up released, and isolate problematic changes.
Eventually, mastering your instruments usually means going past default options and shortcuts — it’s about establishing an personal familiarity with your enhancement environment making sure that when issues occur, you’re not shed at midnight. The higher you recognize your instruments, the greater time you may spend solving the particular trouble rather than fumbling via the method.
Reproduce the trouble
Just about the most essential — and sometimes disregarded — methods in powerful debugging is reproducing the condition. Prior to leaping into your code or earning guesses, builders have to have to make a regular setting or situation in which the bug reliably appears. Without reproducibility, correcting a bug will become a video game of possibility, usually leading to squandered time and fragile code alterations.
The first step in reproducing a dilemma is collecting as much context as feasible. Talk to queries like: What actions brought about The problem? Which environment was it in — progress, staging, or creation? Are there any logs, screenshots, or error messages? The greater detail you have got, the less complicated it gets to be to isolate the precise situations under which the bug takes place.
When you finally’ve gathered sufficient information and facts, seek to recreate the challenge in your local natural environment. This could necessarily mean inputting precisely the same information, simulating similar consumer interactions, or mimicking procedure states. If The problem seems intermittently, contemplate crafting automated checks that replicate the edge scenarios or state transitions included. These checks not merely assistance expose the trouble but also avert regressions in the future.
From time to time, The difficulty could be environment-precise — it might come about only on sure operating programs, browsers, or less than particular configurations. Making use of instruments like Digital machines, containerization (e.g., Docker), or cross-browser testing platforms could be instrumental in replicating these bugs.
Reproducing the problem isn’t just a step — it’s a attitude. It necessitates patience, observation, plus a methodical solution. But when you can constantly recreate the bug, you happen to be already halfway to fixing it. Using a reproducible circumstance, You may use your debugging tools much more efficiently, examination probable fixes securely, and converse extra Evidently together with your crew or end users. It turns an summary grievance into a concrete problem — Which’s wherever builders prosper.
Read and Comprehend the Error Messages
Error messages are frequently the most beneficial clues a developer has when a little something goes Incorrect. As opposed to observing them as discouraging interruptions, builders need to study to deal with error messages as direct communications from your method. They frequently tell you exactly what transpired, wherever it took place, and often even why it occurred — if you know how to interpret them.
Start by reading the information thoroughly As well as in total. Numerous developers, specially when underneath time force, glance at the primary line and right away begin making assumptions. But further inside the error stack or logs may perhaps lie the genuine root result in. Don’t just copy and paste mistake messages into search engines like google and yahoo — read through and realize them initial.
Crack the error down into parts. Can it be a syntax error, a runtime exception, or simply a logic mistake? Does it place to a particular file and line number? What module or purpose triggered it? These thoughts can guidebook your investigation and point you towards the responsible code.
It’s also handy to comprehend the terminology of the programming language or framework you’re employing. Mistake messages in languages like Python, JavaScript, or Java usually follow predictable designs, and learning to recognize these can drastically accelerate your debugging system.
Some problems are imprecise or generic, and in People conditions, it’s important to look at the context during which the mistake occurred. Check out related log entries, input values, and up to date changes inside the codebase.
Don’t forget compiler or linter warnings either. These typically precede larger sized problems and supply hints about potential bugs.
Eventually, mistake messages are not your enemies—they’re your guides. Understanding to interpret them effectively turns chaos into clarity, assisting you pinpoint difficulties faster, minimize debugging time, and turn into a extra efficient and assured developer.
Use Logging Properly
Logging is One of the more powerful resources within a developer’s debugging toolkit. When utilised proficiently, it offers serious-time insights into how an software behaves, aiding you recognize what’s occurring beneath the hood while not having to pause execution or action in the code line by line.
A very good logging strategy starts with understanding what to log and at what level. Typical logging ranges contain DEBUG, Data, Alert, ERROR, and Deadly. Use DEBUG for thorough diagnostic facts for the duration of progress, Details for typical situations (like thriving get started-ups), Alert for probable troubles that don’t split the appliance, ERROR for precise problems, and Lethal in the event the technique can’t keep on.
Keep away from flooding your logs with extreme or irrelevant information. An excessive amount of logging can obscure essential messages and slow down your procedure. Focus on vital gatherings, condition modifications, enter/output values, and significant determination points in your code.
Structure your log messages clearly and consistently. Include things like context, for instance timestamps, request IDs, and performance names, so it’s simpler to trace troubles in distributed devices or multi-threaded environments. Structured logging (e.g., JSON logs) might make it even easier to parse and filter logs programmatically.
All through debugging, logs Allow you to track how variables evolve, what ailments are satisfied, and what branches of logic are executed—all without halting the program. They’re especially precious in creation environments where stepping by way of code isn’t probable.
Additionally, use logging frameworks and resources (like Log4j, Winston, or Python’s logging module) that aid log rotation, filtering, and integration with monitoring dashboards.
In the long run, sensible logging is about equilibrium and clarity. Having a very well-thought-out logging tactic, you are able to lessen the time it takes to spot issues, obtain further visibility into your programs, and Enhance the overall maintainability and dependability within your code.
Consider Like a Detective
Debugging is not simply a technical process—it is a form of investigation. To efficiently detect and resolve bugs, builders have to approach the method just like a detective resolving a secret. This state of mind will help stop working elaborate concerns into workable sections and abide by clues logically to uncover the root lead to.
Start out by gathering evidence. Look at the indicators of the trouble: mistake messages, incorrect output, or effectiveness challenges. Much like a detective surveys against the law scene, obtain just as much applicable information as you are able to with out jumping to conclusions. Use logs, examination situations, and consumer studies to piece collectively a clear image of what’s taking place.
Up coming, kind hypotheses. Check with you: What may very well be resulting in this habits? Have any improvements not long ago been designed to your codebase? Has this challenge transpired ahead of beneath equivalent situations? The goal should be to slender down options and discover likely culprits.
Then, check your theories systematically. Attempt to recreate the problem inside of a managed surroundings. In the event you suspect a selected purpose or element, isolate it and verify if The difficulty persists. Just like a detective conducting interviews, inquire your code questions and Enable the final results lead you nearer to the reality.
Spend shut awareness to tiny details. Bugs normally conceal in the the very least anticipated sites—just like a missing semicolon, an off-by-just one error, or a race affliction. Be comprehensive and patient, resisting the urge to patch The difficulty without having fully knowledge it. Short-term fixes may perhaps conceal the actual issue, just for it to resurface later.
And lastly, maintain notes on That which you experimented with and discovered. Equally as detectives log their investigations, documenting your debugging procedure can help save time for potential challenges and aid Some others comprehend your reasoning.
By imagining like a detective, builders can sharpen their analytical competencies, strategy challenges methodically, and turn out to be simpler at uncovering concealed issues in intricate units.
Compose Assessments
Crafting tests is one of the best tips on how to enhance your click here debugging expertise and Over-all development efficiency. Exams not merely assistance capture bugs early but additionally serve as a safety Internet that offers you confidence when creating adjustments to the codebase. A very well-analyzed software is much easier to debug because it allows you to pinpoint precisely in which and when a challenge happens.
Get started with device assessments, which center on individual functions or modules. These small, isolated tests can rapidly reveal regardless of whether a particular piece of logic is Doing work as predicted. Every time a take a look at fails, you promptly know wherever to glimpse, significantly decreasing time invested debugging. Unit tests are especially practical for catching regression bugs—challenges that reappear just after Beforehand staying fastened.
Following, integrate integration tests and end-to-end checks into your workflow. These enable be sure that several portions of your application function with each other smoothly. They’re significantly handy for catching bugs that come about in intricate techniques with multiple factors or companies interacting. If one thing breaks, your exams can tell you which Element of the pipeline unsuccessful and beneath what circumstances.
Crafting exams also forces you to Consider critically about your code. To check a function properly, you require to grasp its inputs, predicted outputs, and edge circumstances. This level of comprehension Normally potential customers to higher code composition and fewer bugs.
When debugging a difficulty, composing a failing test that reproduces the bug is usually a strong starting point. Once the exam fails constantly, you can focus on repairing the bug and watch your examination go when the issue is solved. This method makes sure that the same bug doesn’t return Later on.
To put it briefly, creating assessments turns debugging from the irritating guessing match right into a structured and predictable procedure—supporting you capture more bugs, more quickly plus much more reliably.
Take Breaks
When debugging a tricky situation, it’s quick to become immersed in the trouble—observing your monitor for hours, trying Remedy immediately after solution. But Just about the most underrated debugging instruments is actually stepping absent. Having breaks assists you reset your thoughts, lessen irritation, and often see the issue from a new point of view.
When you are far too near the code for much too lengthy, cognitive fatigue sets in. You could start off overlooking evident glitches or misreading code you wrote just several hours previously. With this condition, your brain gets considerably less productive at dilemma-fixing. A short walk, a espresso crack, or simply switching to another task for ten–quarter-hour can refresh your concentration. Quite a few builders report locating the basis of an issue after they've taken the perfect time to disconnect, allowing their subconscious operate while in the track record.
Breaks also aid avoid burnout, Particularly throughout lengthier debugging sessions. Sitting down before a display, mentally trapped, is not simply unproductive but in addition draining. Stepping away helps you to return with renewed Electricity as well as a clearer state of mind. You may perhaps instantly observe a missing semicolon, a logic flaw, or maybe a misplaced variable that eluded you in advance of.
Should you’re stuck, a great general guideline is usually to set a timer—debug actively for forty five–sixty minutes, then take a five–ten moment split. Use that time to maneuver about, extend, or do some thing unrelated to code. It could truly feel counterintuitive, Specially under restricted deadlines, but it in fact results in speedier and more effective debugging In the long term.
In brief, getting breaks is not a sign of weak spot—it’s a smart approach. It gives your brain Place to breathe, increases your viewpoint, and allows you avoid the tunnel vision That always blocks your progress. Debugging can be a psychological puzzle, and rest is a component of resolving it.
Discover From Every single Bug
Every bug you come across is a lot more than simply A brief setback—It is really an opportunity to expand for a developer. Regardless of whether it’s a syntax mistake, a logic flaw, or simply a deep architectural concern, every one can instruct you anything important in the event you make the effort to replicate and evaluate what went Completely wrong.
Start by asking by yourself a handful of key concerns after the bug is solved: What brought about it? Why did it go unnoticed? Could it are actually caught earlier with better methods like unit testing, code testimonials, or logging? The solutions generally expose blind places as part of your workflow or being familiar with and assist you build stronger coding habits moving ahead.
Documenting bugs will also be an excellent habit. Keep a developer journal or maintain a log in which you Take note down bugs you’ve encountered, the way you solved them, and Whatever you uncovered. After a while, you’ll start to see patterns—recurring issues or typical mistakes—that you can proactively avoid.
In team environments, sharing Anything you've figured out from the bug along with your peers is usually Primarily highly effective. No matter whether it’s by way of a Slack message, a brief publish-up, or a quick knowledge-sharing session, encouraging Some others stay away from the exact same difficulty boosts staff efficiency and cultivates a much better Finding out tradition.
More importantly, viewing bugs as classes shifts your state of mind from frustration to curiosity. In place of dreading bugs, you’ll commence appreciating them as critical portions of your advancement journey. After all, many of the very best builders aren't those who compose fantastic code, but individuals who constantly study from their blunders.
Eventually, Each and every bug you take care of adds a completely new layer for your talent set. So up coming time you squash a bug, have a moment to mirror—you’ll occur away a smarter, additional capable developer on account of it.
Summary
Strengthening your debugging competencies requires time, exercise, and patience — nevertheless the payoff is large. It makes you a far more efficient, assured, and able developer. The subsequent time you might be knee-deep inside a mysterious bug, recall: debugging isn’t a chore — it’s a possibility to become far better at That which you do.